Python programlama dili ile Kütüphane takip uygulaması yazmak için aşağıdaki adımları takip edebiliriz:
- Veri Modeli Oluşturma: Öğrenciler ve kitaplar için veri modelleri oluşturacağız.
- İşlevler Tanımlama: Kitap ödünç alma, kitap iade etme ve süresi geçen kitapları kontrol etme işlevlerini tanımlayacağız.
- Bildirim Sistemi: Süresi geçen kitaplar için öğrencilere bildirim gönderme işlevini ekleyeceğiz.
Ayrıca Tkinter kullanarak bu uygulamanın grafiksel bir arayüzünü (GUI) oluşturabiliriz. Ekran görüntüsü aşağıda gösterilmektedir.
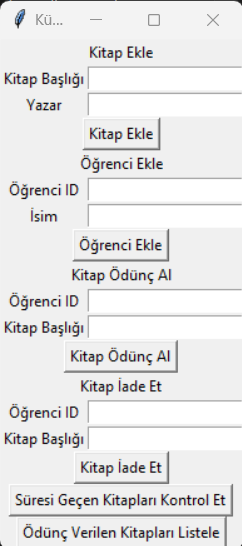
Kod bloğu aşağıda verilmektedir.
import tkinter as tk
from tkinter import messagebox
from datetime import datetime, timedelta
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
self.is_borrowed = False
class Student:
def __init__(self, student_id, name):
self.student_id = student_id
self.name = name
self.borrowed_books = {}
class Library:
def __init__(self):
self.books = []
self.students = []
self.borrowed_books = {}
def add_book(self, book):
self.books.append(book)
def add_student(self, student):
self.students.append(student)
def borrow_book(self, student_id, book_title):
student = next((s for s in self.students if s.student_id == student_id), None)
book = next((b for b in self.books if b.title == book_title and not b.is_borrowed), None)
if student and book:
book.is_borrowed = True
due_date = datetime.now() + timedelta(days=30)
student.borrowed_books[book_title] = due_date
self.borrowed_books[book_title] = (student_id, due_date)
messagebox.showinfo("Başarılı", f"{book_title} kitabı {student.name} adlı öğrenciye {due_date.strftime('%Y-%m-%d')} tarihine kadar ödünç verildi.")
else:
messagebox.showerror("Hata", "Kitap mevcut değil veya öğrenci bulunamadı.")
def return_book(self, student_id, book_title):
student = next((s for s in self.students if s.student_id == student_id), None)
if student and book_title in student.borrowed_books:
del student.borrowed_books[book_title]
book = next((b for b in self.books if b.title == book_title), None)
if book:
book.is_borrowed = False
del self.borrowed_books[book_title]
messagebox.showinfo("Başarılı", f"{book_title} kitabı iade edildi.")
else:
messagebox.showerror("Hata", "Kitap veya öğrenci bulunamadı.")
def check_overdue_books(self):
now = datetime.now()
overdue_books = [(title, info) for title, info in self.borrowed_books.items() if info[1] < now]
if overdue_books:
message = "\n".join([f"{title}: {due_date.strftime('%Y-%m-%d')} teslim tarihi geçmiş" for title, (student_id, due_date) in overdue_books])
messagebox.showwarning("Süresi Geçmiş Kitaplar", message)
else:
messagebox.showinfo("Bilgi", "Süresi geçmiş kitap yok.")
def list_borrowed_books(self):
borrowed_books_info = [f"{title} - Öğrenci ID: {info[0]}, Teslim Tarihi: {info[1].strftime('%Y-%m-%d')}" for title, info in self.borrowed_books.items()]
if borrowed_books_info:
message = "\n".join(borrowed_books_info)
else:
message = "Ödünç verilen kitap yok."
messagebox.showinfo("Ödünç Verilen Kitaplar", message)
library = Library()
# Tkinter GUI
root = tk.Tk()
root.title("Kütüphane Takip Uygulaması")
def add_book():
title = book_title_entry.get()
author = book_author_entry.get()
if title and author:
book = Book(title, author)
library.add_book(book)
messagebox.showinfo("Başarılı", "Kitap eklendi.")
else:
messagebox.showerror("Hata", "Lütfen tüm bilgileri doldurun.")
def add_student():
student_id = student_id_entry.get()
name = student_name_entry.get()
if student_id and name:
student = Student(student_id, name)
library.add_student(student)
messagebox.showinfo("Başarılı", "Öğrenci eklendi.")
else:
messagebox.showerror("Hata", "Lütfen tüm bilgileri doldurun.")
def borrow_book():
student_id = borrow_student_id_entry.get()
book_title = borrow_book_title_entry.get()
if student_id and book_title:
library.borrow_book(student_id, book_title)
else:
messagebox.showerror("Hata", "Lütfen tüm bilgileri doldurun.")
def return_book():
student_id = return_student_id_entry.get()
book_title = return_book_title_entry.get()
if student_id and book_title:
library.return_book(student_id, book_title)
else:
messagebox.showerror("Hata", "Lütfen tüm bilgileri doldurun.")
def check_overdue_books():
library.check_overdue_books()
def list_borrowed_books():
library.list_borrowed_books()
# Kitap ekleme
tk.Label(root, text="Kitap Ekle").grid(row=0, column=0, columnspan=2)
tk.Label(root, text="Kitap Başlığı").grid(row=1, column=0)
book_title_entry = tk.Entry(root)
book_title_entry.grid(row=1, column=1)
tk.Label(root, text="Yazar").grid(row=2, column=0)
book_author_entry = tk.Entry(root)
book_author_entry.grid(row=2, column=1)
tk.Button(root, text="Kitap Ekle", command=add_book).grid(row=3, column=0, columnspan=2)
# Öğrenci ekleme
tk.Label(root, text="Öğrenci Ekle").grid(row=4, column=0, columnspan=2)
tk.Label(root, text="Öğrenci ID").grid(row=5, column=0)
student_id_entry = tk.Entry(root)
student_id_entry.grid(row=5, column=1)
tk.Label(root, text="İsim").grid(row=6, column=0)
student_name_entry = tk.Entry(root)
student_name_entry.grid(row=6, column=1)
tk.Button(root, text="Öğrenci Ekle", command=add_student).grid(row=7, column=0, columnspan=2)
# Kitap ödünç alma
tk.Label(root, text="Kitap Ödünç Al").grid(row=8, column=0, columnspan=2)
tk.Label(root, text="Öğrenci ID").grid(row=9, column=0)
borrow_student_id_entry = tk.Entry(root)
borrow_student_id_entry.grid(row=9, column=1)
tk.Label(root, text="Kitap Başlığı").grid(row=10, column=0)
borrow_book_title_entry = tk.Entry(root)
borrow_book_title_entry.grid(row=10, column=1)
tk.Button(root, text="Kitap Ödünç Al", command=borrow_book).grid(row=11, column=0, columnspan=2)
# Kitap iade etme
tk.Label(root, text="Kitap İade Et").grid(row=12, column=0, columnspan=2)
tk.Label(root, text="Öğrenci ID").grid(row=13, column=0)
return_student_id_entry = tk.Entry(root)
return_student_id_entry.grid(row=13, column=1)
tk.Label(root, text="Kitap Başlığı").grid(row=14, column=0)
return_book_title_entry = tk.Entry(root)
return_book_title_entry.grid(row=14, column=1)
tk.Button(root, text="Kitap İade Et", command=return_book).grid(row=15, column=0, columnspan=2)
# Süresi geçen kitapları kontrol etme
tk.Button(root, text="Süresi Geçen Kitapları Kontrol Et", command=check_overdue_books).grid(row=16, column=0, columnspan=2)
# Ödünç verilen kitapları listeleme
tk.Button(root, text="Ödünç Verilen Kitapları Listele", command=list_borrowed_books).grid(row=17, column=0, columnspan=2)
root.mainloop()
Bu örnekte Tkinter ile arayüzünü oluşturduğumuz Kütüphane Takip programını yaptık. Burada herhangi bir veritabanı kullanılmamıştır. Verilerin sürekli olarak saklanması isteniliyorsa veri tabanı kullanılmalıdır.